If you read enough of my posts, you'll probably notice I like to build python bots. They’re quick to code and some simple logic is all you need to start doing cool stuff. I love seeing them live in action interacting with the real world. I wanted to create a Twitter bot that would tweet my stock trading positions from my TD Ameritrade account. I have always been interested in the markets. I studied econ for my undergrad and became a licensed broker in 2016 when I worked as a financial advisor. My passion for markets remained even after I changed industries to be a software developer. I always love when two of my interests intersect, in this case software development and stock markets! This bot is running and active on Twitter, just click here or search for @stephens_log. Scroll down and I'll walk you through the code.
2020
Python, API, Heroku, TD Ameritrade, Twitter
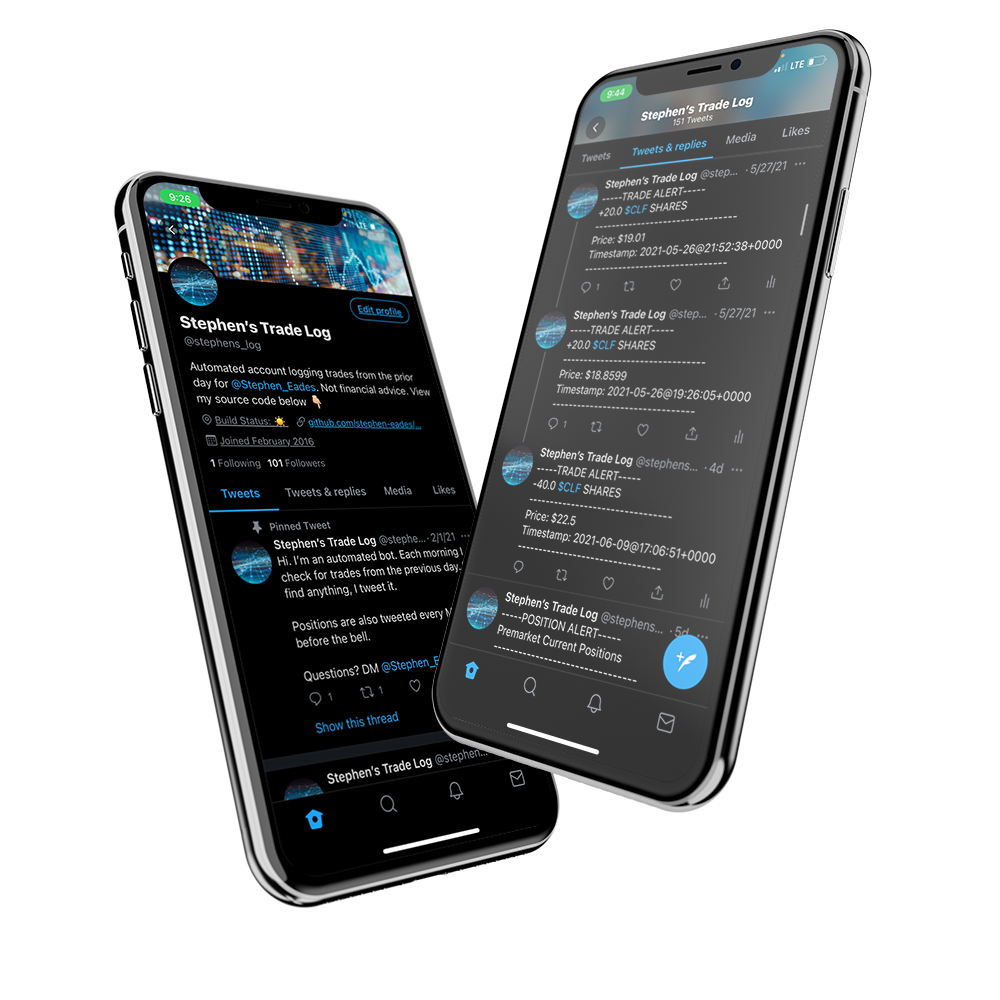
Fancy picture of my bot's twitter page
The first thing I had to do in the code for this bot was authentication. Heroku allows you to set configuration variables that can be used in the code, so you can safely enter your Twitter and TD Ameritrade API keys for use. I also added some local variables to replicate the keys stored in Heroku so I could test my bot locally before deploying.
Authentication variables
Next we can use the API keys to get the access token. Using some if-else statements our bot first checks to see if there is an existing and valid refresh token that can be used to get a new access token. If not, we use a provided auth code to kickoff a new authentication flow. This will then provide us an access token and refresh token which can be stored. Refresh tokens expire every 90 days, so the auth code must be used each quarter to get a new refresh token.
Authentication function
I created two functions that encompass the main flows for each type of tweet my bot sends out. Both are detailed below, and you'll be able to see the helper functions I mention later in this post as well.
1. tweet_trades_from_prior_day - Tweets out each specific position change from the prior day every morning pre-market. After getting an access token using the authentication method, the bot gathers all recent stock or options trades from my Ameritrade account. Then it passes that trading data into a helper function that formats the data into readable tweets. The prepared tweets are then handed off to the final function titled "send_tweets" which does exactly as it's titled. You can also see I pass in the twitter authentication needed to post tweets using Twitter's API.
2. tweet_positions - Tweets the current positions held in the account each morning pre-market. This follows a similar pattern as the first function, however it checks specifically for current positions instead of changes to positions. After authenticating, the position data is handed to a helper function that grabs the ticker symbol of each position and returns it in a simple list. This list of tickers is then formatted and used in the tweet.
Two main functions of the bot
I wanted to also show where exactly I am making these API requests to, and what the data I am getting back looks like before formatting. The resource endpoint is below, where I use my account ID from Ameritrade to request all transactions from my account. I can then check within my code for the most recent transaction from the prior day.
The url to get all transactions for an Ameritrade account
The response data for each transaction is in JSON format and is summarized below. You can see we have plenty of information to go through, and mostly comprised of number type data which is expected as we're dealing with financial information. With a quick glance at this data, you can see the bot now has everything it needs to create a tweet about the trade that occurred.
Each transaction is returned in JSON format
To make the formatting and sending of the tweets easier, I created a Tweet class. Python classes let you handle the data as you would traditionally with object oriented programming. This is nice in our case, as we can treat each Tweet as its own object with unique attributes. The initialization of the class object is standard, with a few assignments requiring the conversion of numbers into string data types.
Python class for a Tweet object and the initialization
With each tweet formatted and organized into a Tweet class, our next step is to send the qualifying tweets out! We authorize with Twitter's API using a handy library called Tweepy which I highly recommend if you want to work with Python and Twitter's API. Some string formatting finishes up the process, where you can see the tweet is sent out using the "update_status" function.
The data for the tweet is ready to be posted to Twitter
The tweet is now live at this point, and available for the world to see! Well, at least available for my bot's followers. You can see in the image below that I also group trades of the same asset into a thread. If you're interested in seeing the code in its entirety, or want to fork the code and get your own Twitter trade bot running, check out the Github repository here!
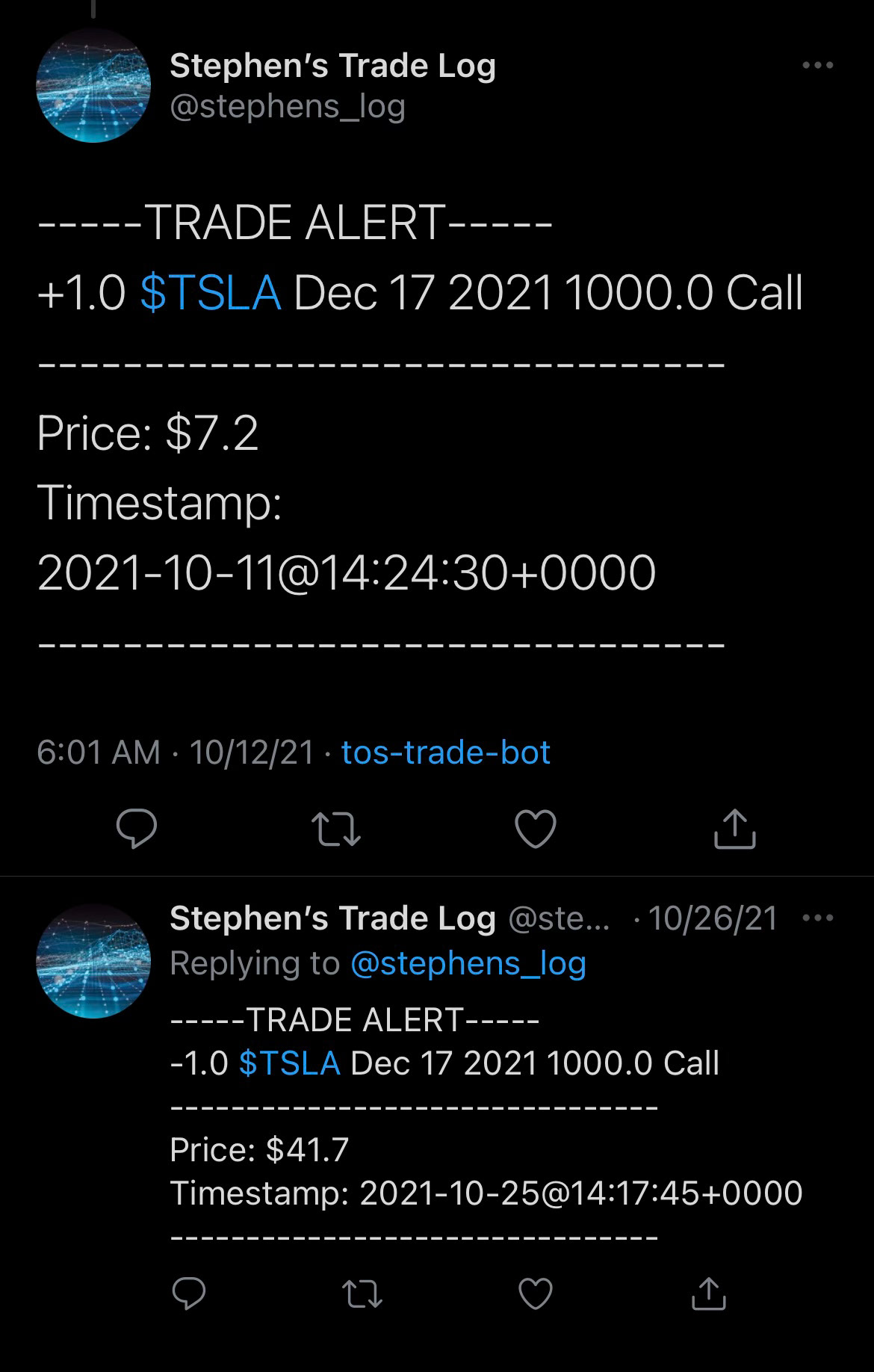
Example thread for an asset